make a binary search tree algorithm in Python
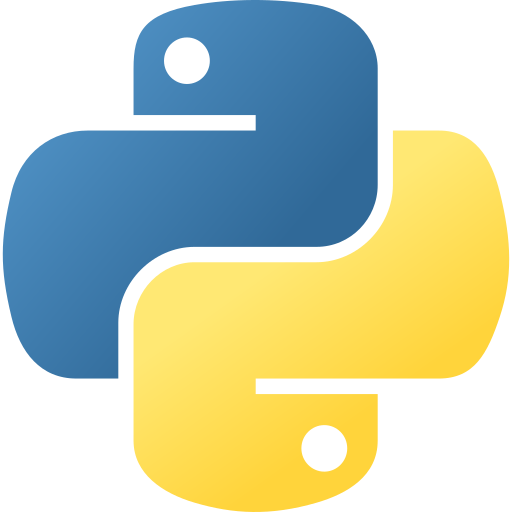
Python
🤖 Code Explanation
def inorder(root):
if root:
inorder(root.left)
print(root.data)
inorder(root.right)
def search(root, key):
if root is None or root.data == key:
return root
if root.data < key:
return search(root.right, key)
return search(root.left, key)
r = Node(50)
insert(r,Node(30))
insert(r,Node(20))
insert(r,Node(40))
insert(r,Node(70))
insert(r,Node(60))
insert(r,Node(80))
inorder(r)
x = search(r, 20
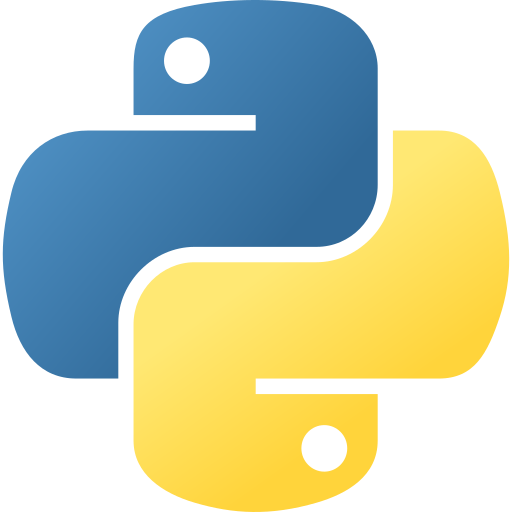
More problems solved in Python
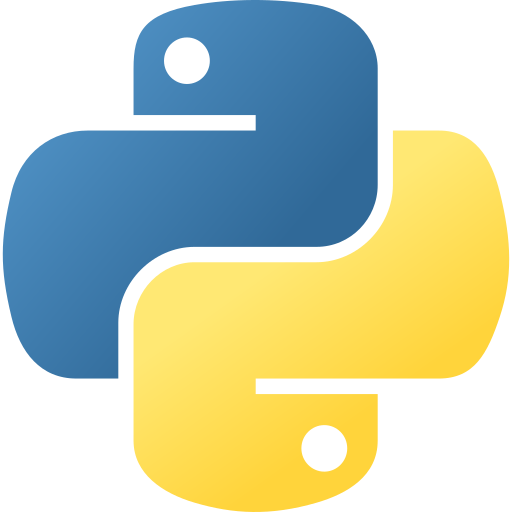
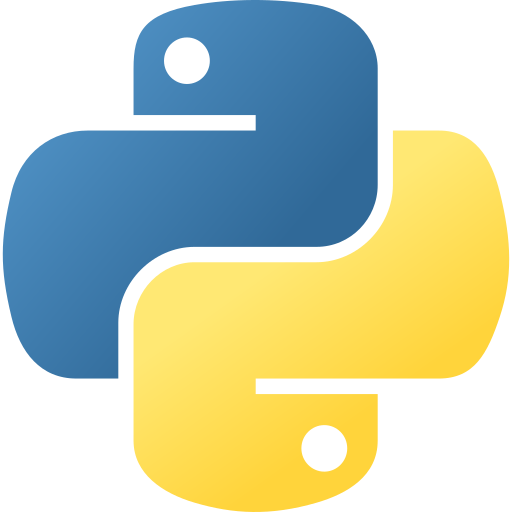
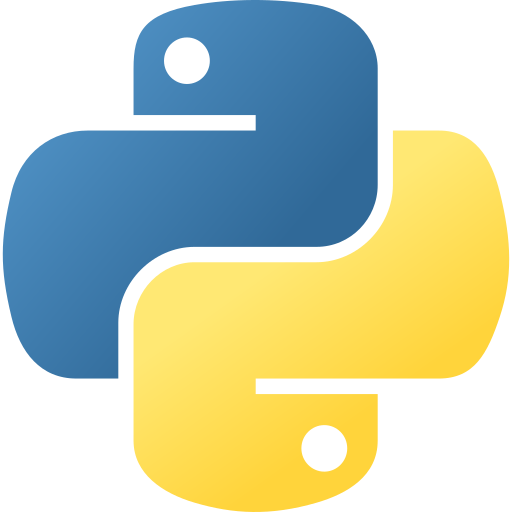
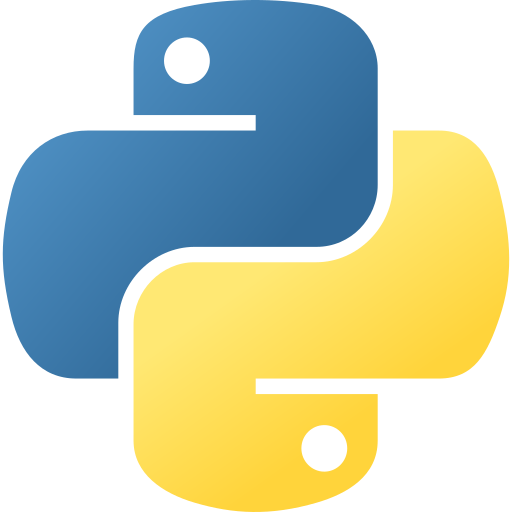
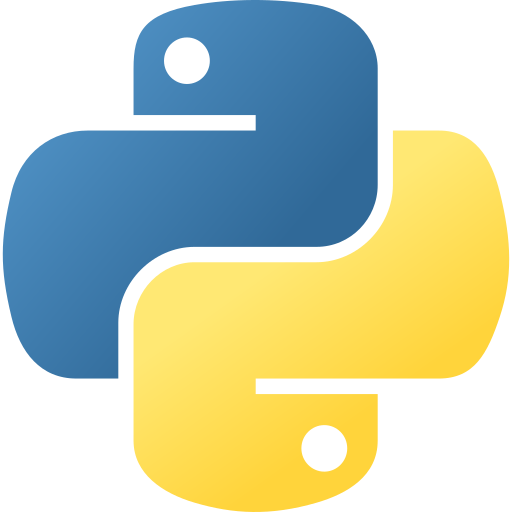
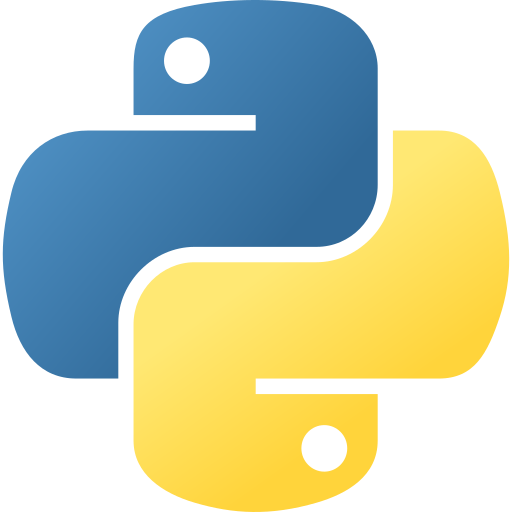
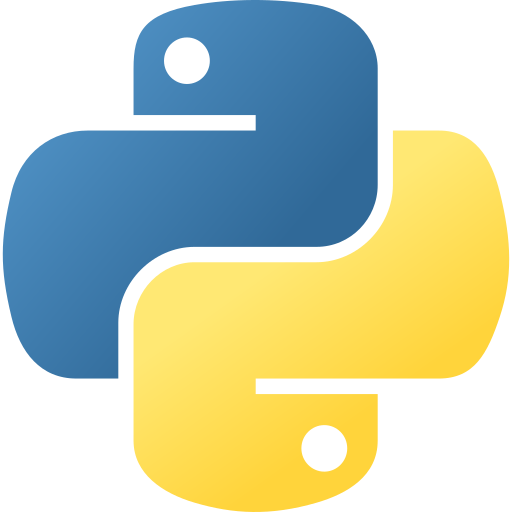
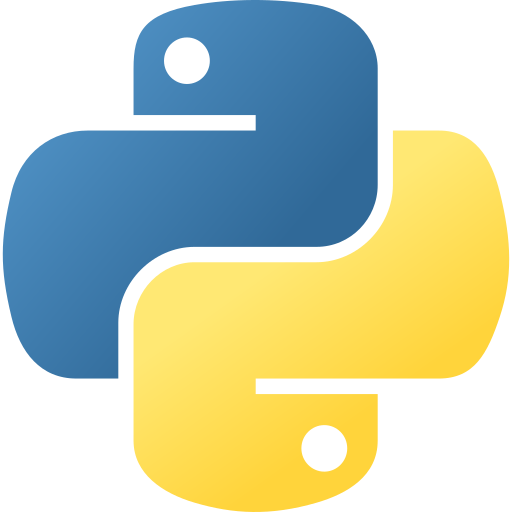
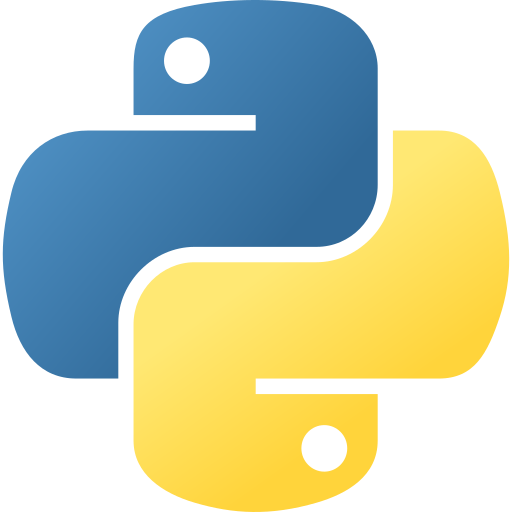
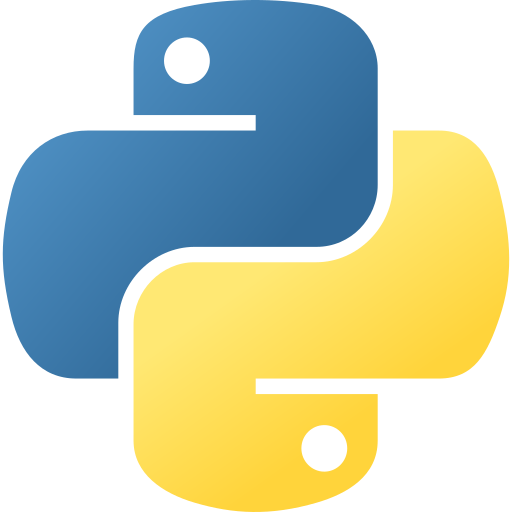
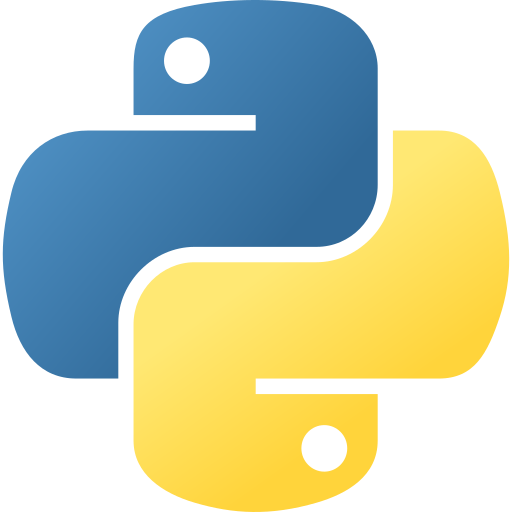
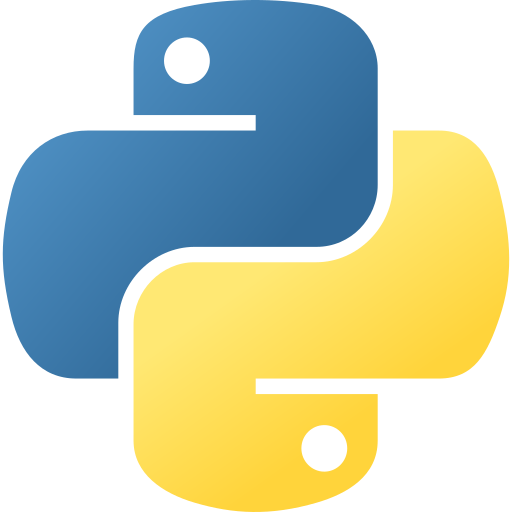
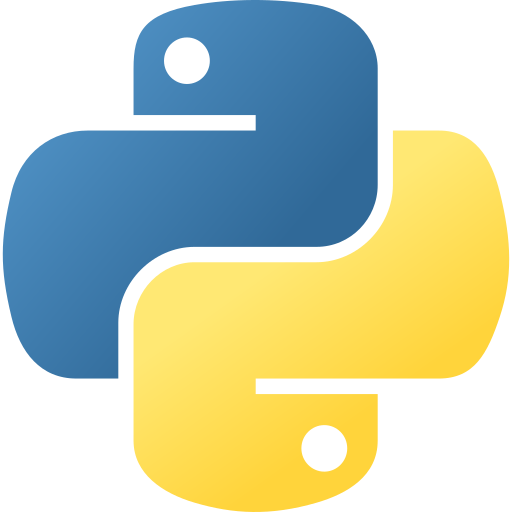
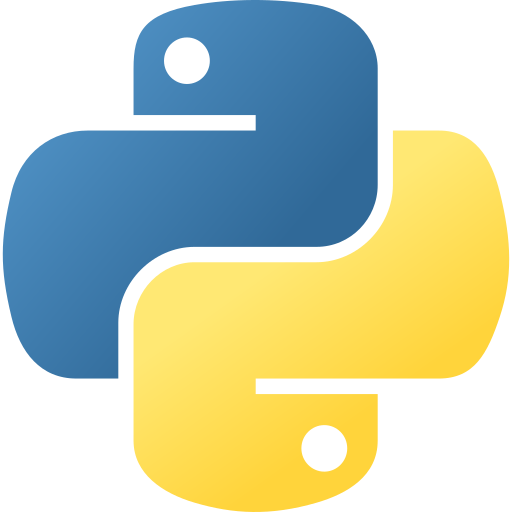
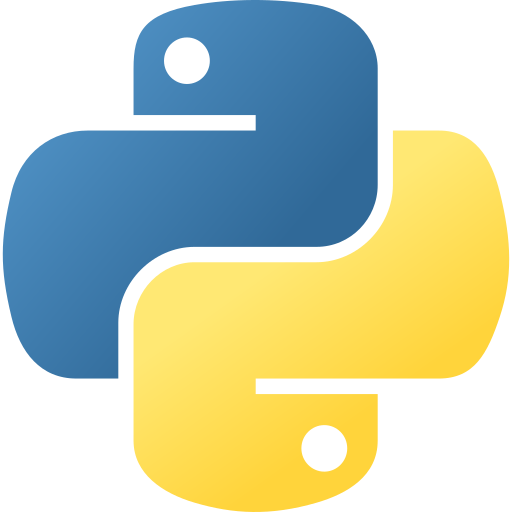
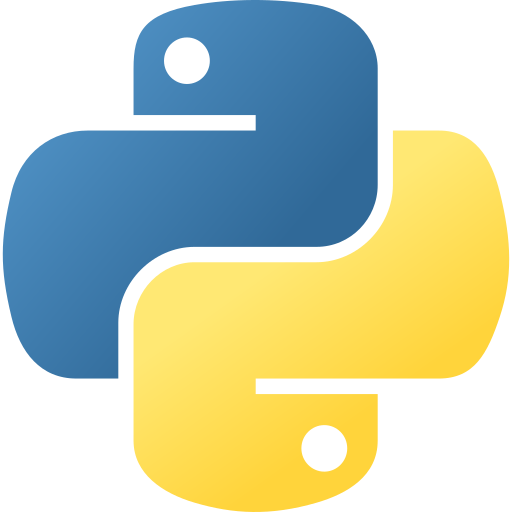
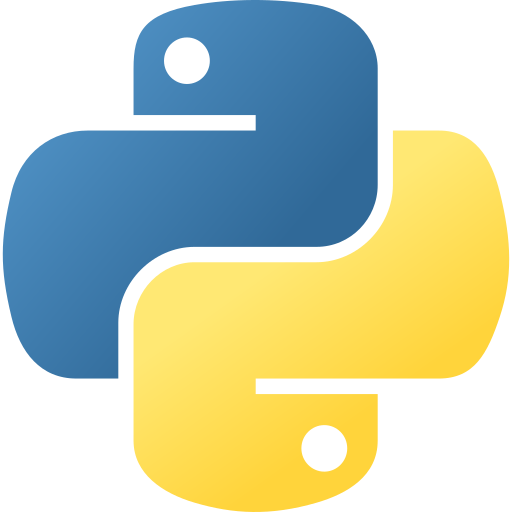
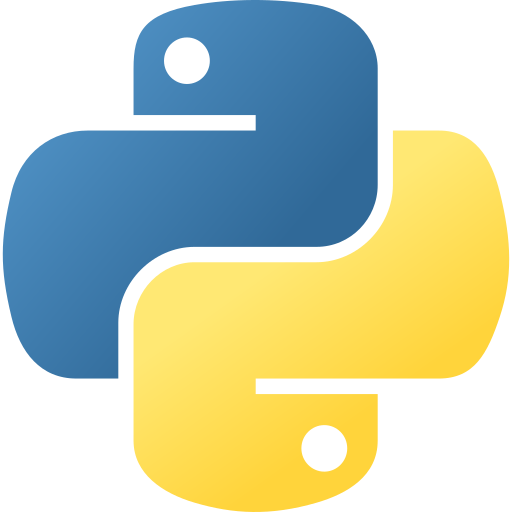
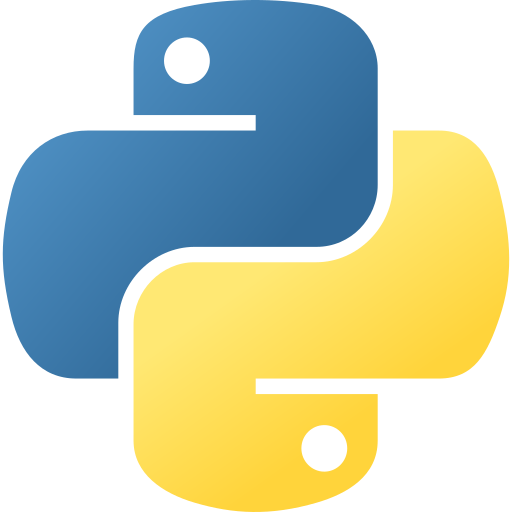